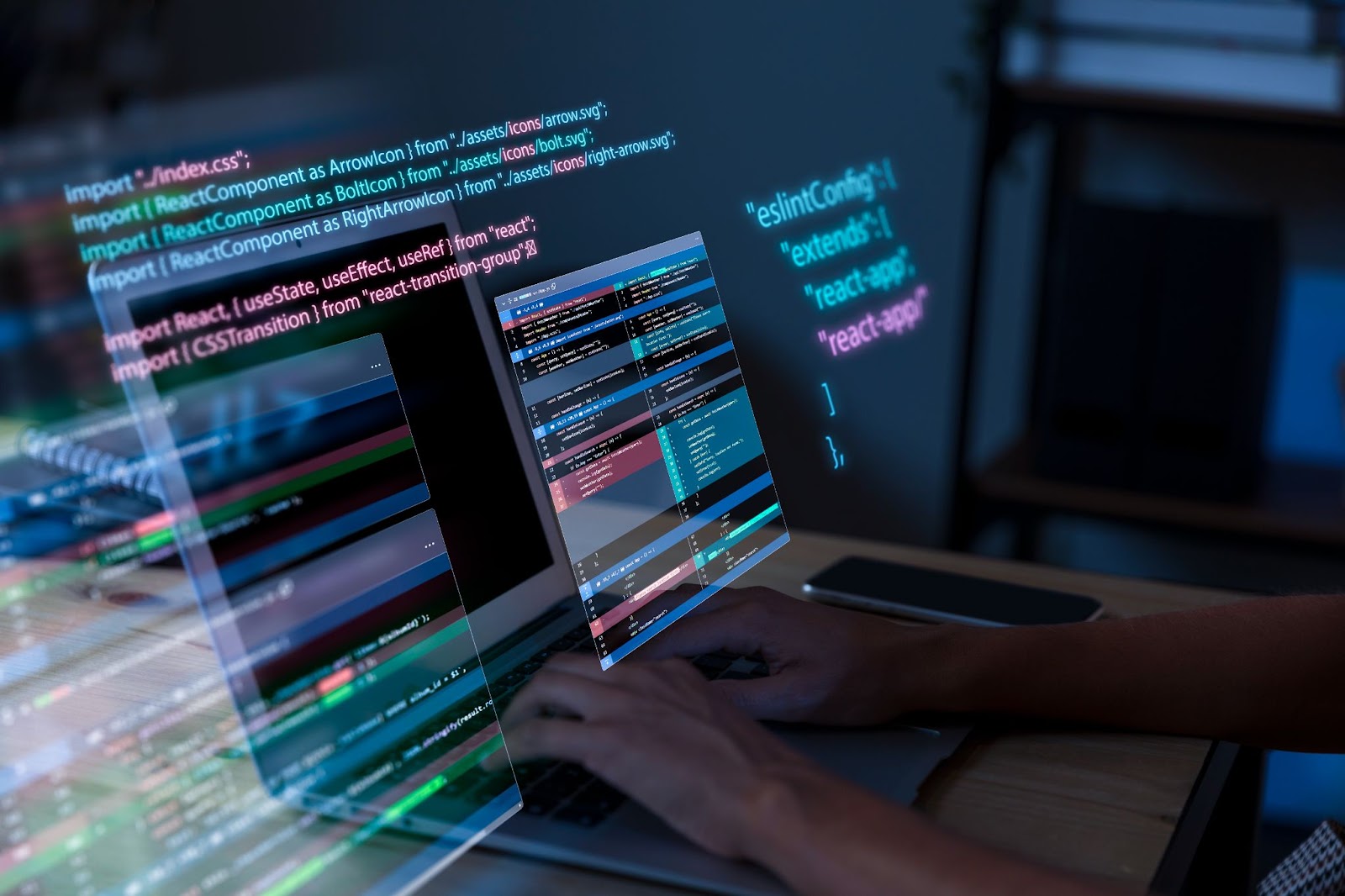
In the ever-evolving realm of cloud computing, AWS Lambda stands out as a true game-changer, completely transforming the landscape of how organizations go about crafting and deploying their applications. Serverless computing, characterized by its event-driven, highly scalable, and cost-efficient attributes, has swiftly risen to prominence. Yet, one must bear in mind that with great power comes an even greater responsibility, particularly in the context of monitoring and logging within a serverless environment.
Within the following discussion, we shall embark on a journey into the domain of optimal AWS Lambda logging practices. Whether you’re a seasoned veteran of Lambda utilization or are just embarking on your expedition into the world of serverless computing, comprehending the art of effective logging proves indispensable. It not only ensures sustained visibility into your applications but also equips you with the means to diagnose issues, fine-tune performance, and uphold security compliance.
Come with us as we embark on an exploration of the core strategies and tools that will empower you to navigate the intricate terrain of AWS Lambda logging. These insights will guarantee that your serverless applications function at their utmost potential.
Understanding AWS Lambda Logging: An Expanded Overview
AWS Lambda represents a revolutionary approach to executing code in the cloud, offering a serverless architecture. This means that developers can run their code without the need to manage or provision servers. The dynamic scalability of AWS Lambda is one of its most impressive features, effortlessly handling anywhere from a minimal number of requests to thousands per second. This flexibility is a game-changer in cloud computing.
Key Advantages of AWS Lambda:
- Serverless Execution: Eliminates the need for server management, simplifying the deployment process;
- Cost-Effective: You only pay for the execution time of your code, not for idle time. This can lead to substantial cost savings;
- Scalability: Automatically adjusts to handle the number of requests, whether it’s just a few or several thousand per second.
AWS Lambda functions are particularly useful for short-duration tasks. A typical use case involves passing a function as an argument to a higher-order function, enabling more complex and dynamic code execution without the overhead of traditional server management.
AWS Lambda Logging: A Deep Dive
AWS Lambda logging is an integral feature that provides an automated monitoring system for all Lambda functions. It employs AWS CloudWatch, a powerful logging and monitoring service, to track the activities and performance of Lambda functions.
Key Features of AWS Lambda Logging:
- Automatic Monitoring: Tracks the performance and execution of Lambda functions without manual intervention;
- Integration with CloudWatch: Offers a comprehensive logging solution using AWS CloudWatch;
- Function Activity Grouping: Enables grouping and categorization of function activities for better organization and analysis;
- Instance-Level Logging: Provides detailed logs for each instance of your function, allowing for in-depth troubleshooting and performance analysis.
Implementing Logging in AWS Lambda with Python
Logging is an essential aspect of monitoring and debugging in AWS Lambda functions. To effectively implement logging in Python, here’s a comprehensive guide.
Creating the Lambda Function for Logging:
Begin by importing necessary modules. In this case, os is required.
Define the lambda_handler function, which is the entry point for Lambda executions.
Code Structure:
import os
def lambda_handler(event, context):
# Log Environment Variables
print('Environment Variables:')
print(os.environ)
# Log Event Data
print('Event Data:')
print(event)
Key Components of the Logging Code:
- Environment Variables: Use print(os.environ) to log the environment variables. This step is crucial for understanding the Lambda function’s context;
- Event Data: Log the event object to capture the input received by the Lambda function. This information is critical for debugging and understanding the function’s operation.
Understanding the Log Format:
The log starts with a Start Request line, indicating the beginning of a Lambda invocation.
Following this, the Environment Variables and Event Data are logged, providing insight into the function’s operational context and input. The log concludes with an End Request line and a Report section, which includes critical performance metrics.
Key Metrics in the Log Report:
- RequestId: A unique identifier for each invocation, aiding in tracking and debugging specific requests;
- Duration: Time taken by the Lambda function to process the event;
- Billed Duration: Time billed for the invocation, rounded up to the nearest 100ms;
- Memory Size: The memory allocated to the Lambda function;
- Max Memory Used: The peak memory usage during the function’s execution;
- Init Duration: Time taken to initialize the function on the first request, including loading libraries and other setup tasks;
- XRAY TraceId and SegmentId: For AWS X-Ray traced requests, these IDs provide detailed tracing information;
- Sampled: Indicates whether the request was sampled for tracing purposes.
Mastering AWS Command Line Interface (CLI): A Comprehensive Guide
The AWS Command Line Interface (CLI) is a powerful, open-source tool designed to enable seamless interaction and automation of AWS services directly from your command line environment. This tool is a gateway for developers and IT professionals to efficiently manage their AWS services.
Getting Started with AWS CLI
Installation of AWS CLI Version 2
- Begin by installing the latest version of AWS CLI. AWS CLI Version 2 is the most up-to-date version, offering improved features and compatibility;
- Detailed instructions for various operating systems can be found on the AWS website.
Configuration for Optimal Use
- Once installed, configure AWS CLI by entering your AWS credentials and setting your preferred region and output format;
- This configuration process simplifies subsequent AWS service commands, making your workflow more efficient.
Utilizing AWS CLI for Log Retrieval
Retrieving Logs from CloudWatch Using AWS CLI
AWS CLI is particularly useful for retrieving logs from AWS CloudWatch, a monitoring and observability service.
To fetch logs, specific commands need to be executed. For example, to retrieve an ID, use the following command structure:
aws lambda invoke --function-name [function-name] [output-file] --log-type Tail
Replace [function-name] with the name of your Lambda function and [output-file] with your desired output file’s name.
Understanding the Output
The output of this command will provide essential information such as status code, log result, and executed version.
An example output looks like this:
{
"StatusCode": 200,
"LogResult": "Encoded log data...",
"ExecutedVersion": "$LATEST"
}
Here, StatusCode indicates the success of the operation, LogResult contains the log data (usually encoded), and ExecutedVersion shows the version of the Lambda function that was executed.
Extracting AWS Lambda Logs: A Detailed Guide
To retrieve AWS Lambda logs effectively, use the AWS CLI with specific commands. This involves invoking the function and requesting log outputs in a particular format.
Command Syntax: Start by using aws lambda invoke, specifying the function name (e.g., my-function) and an output file (e.g., out). To extract logs, add –log-type Tail and format the output using –query ‘LogResult’ –output text. Then, decode the base64 output using base64 -d.
Understanding the Output
The output provides a wealth of information, including the request ID, session tokens, and trace IDs.
- Key Components:
- Start Line: Indicates the beginning of the request, showing the request ID and version;
- Session Information: Contains the AWS session token and Amazon Trace ID, crucial for tracking and security purposes;
- End Line: Marks the completion of the request, repeating the request ID for easy correlation;
- Report Line: Provides vital metrics like execution duration, billed duration, memory allocation, and actual memory usage.
- Tips for Analysis:
- Pay attention to the duration and memory usage to optimize function performance;
- Use the trace ID for debugging and tracing the request path in distributed systems.
Using CLI Binary Format for Advanced Log Retrieval
This method allows for more nuanced control and processing of logs.
- Step-by-Step Process:
- Invoke the Function: Use aws lambda invoke with –cli-binary-format raw-in-base64-out to handle the payload effectively;
- Payload Handling: Include a JSON payload with your key-value pairs;
- Output Processing: Use sed to clean the output file, removing any unwanted characters;
- Pause Execution: Employ sleep to delay the script, ensuring logs are fully generated;
- Retrieve Logs: Use aws logs get-log-events, specifying the log group and stream names. Control the output using –limit.
- Recommendations for Effective Use:
- Modify the payload as per your function’s needs;
- Adjust the sleep duration based on your function’s execution time;
- Use the limit parameter to control the amount of log data retrieved, focusing on recent and relevant events.
Modifying File Permissions and Running Scripts in macOS and Linux
Adjusting File Permissions
To ensure the proper execution of shell scripts in macOS and Linux, it’s crucial to set the correct file permissions. For instance, to modify the permissions of a script named get-logs.sh, one can use the chmod command:
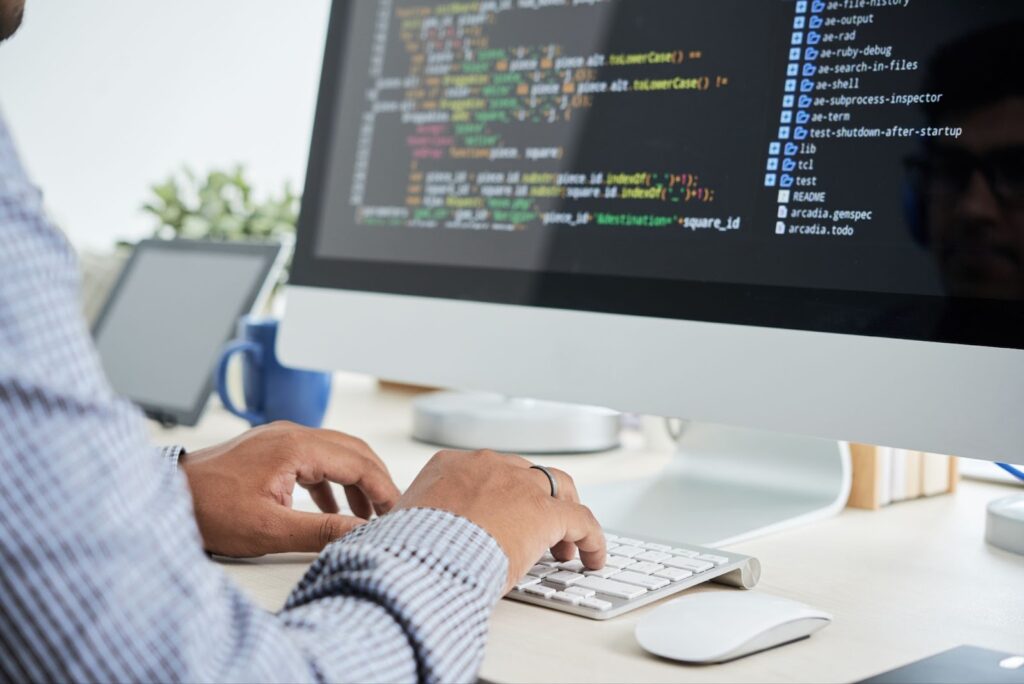
- Command Explanation: The chmod -R 755 get-logs.sh command modifies the file permissions, setting the read, write, and execute permissions appropriately for the user, and read and execute permissions for the group and others.
Steps to Follow:
- Open Terminal.
- Navigate to the directory containing get-logs.sh;
- Enter chmod -R 755 get-logs.sh and press Enter;
- Executing the Script;
- Once the permissions are set, executing the script is straightforward:
- Run the Script: Simply type ./get-logs.sh in the terminal and press Enter;
- Expected Output: After running the script, the terminal should display a JSON output indicating the status and details of the execution.
Understanding the Output
The output typically consists of a JSON formatted response, which includes several key components:
- Status Code: Shows 200, indicating successful execution;
- Executed Version: Indicates the version of the script or function executed, usually $LATEST;
- The output also includes an events array, providing detailed logs:
- Each event contains a timestamp, message, and ingestionTime.
- Types of Messages:
- Start and End Requests: Indicate the beginning and end of a process;
- Info Logs: Provide insights into the environment variables and other execution details;
- Report Details: Include metrics like execution duration, memory usage, and billing information.
Optimizing Log Ingestion Costs in AWS Lambda
Are you looking to optimize log ingestion costs in your AWS Lambda functions? Excessive logging can quickly add up, leading to higher expenses. In this comprehensive guide, we’ll explore effective strategies to manage log data efficiently, reduce costs, and improve the overall monitoring and debugging experience.
1. Utilize Logging Libraries with Severity Levels
To minimize the volume of log data generated by your AWS Lambda functions, consider implementing logging libraries that support severity levels. Here’s how you can set this up:
Serverless.yml Configuration:
Define log levels based on your environment (e.g., ‘prod’ and ‘staging’).
Set the default log level to ‘debug’ for non-production environments and ‘info’ for production and staging environments.
custom:
logLevelMap:
prod: info
staging: info
logLevel: ${self:custom.logLevelMap.${opt:stage}, 'debug'}
provider:
environment:
LOG_LEVEL: ${self:custom.logLevel}
Logger.ts Implementation:
Create a logger using your chosen logging library.
Set the log level based on the environment variable ‘LOG_LEVEL.’
const logger = someLoggerLib.createLogger({ level: process.env.LOG_LEVEL || 'info' });
This approach allows you to adjust log levels dynamically, ensuring that you only capture the necessary information for debugging and monitoring in different environments.
2. Optimize Log Retention
Managing log retention is crucial to avoid storing unnecessary log data indefinitely. AWS allows you to configure log retention in days. Set it to an appropriate value, such as 30 days, to strike a balance between historical data preservation and cost control. Add the following parameter to your serverless.yml file:
provider:
logRetentionInDays: 30
By setting a maximum retention period, you can avoid accumulating logs that are no longer relevant.
3. Log as JSON for Efficient Parsing
Logging data in JSON format not only makes it more readable but also facilitates efficient parsing and filtering. CloudWatch appreciates JSON logs, making it a powerful choice for AWS Lambda logging. Here’s an example of how to log in JSON format:
{
"level": "info",
"message": "Data ingest completed",
"data": {
"items": 42,
"failures": 7
}
}
By structuring your logs in this way, you can easily filter them based on specific attributes like message content, making debugging and analysis more precise.
4. Simplify Logger Configuration with Winston
For Node.js Lambda functions, the Winston library is a popular choice for logging. Simplify your logger configuration with these steps:
- Set the log level dynamically using an environment variable;
- Format logs as JSON for better readability and parsing;
- Include the request ID in each log message for tracing purposes;
- Optionally attach additional data for context.
{
"level": "info",
"message": "Data ingest completed",
"data": {
"items": 42,
"failures": 7
}
}
By following these practices, you can streamline your logging setup, reduce costs associated with excessive log data, and enhance the effectiveness of monitoring and debugging in your AWS Lambda functions. Read about the pivotal role of cloud security in safeguarding your data and operations. Learn why cloud security is important for your digital journey.
Conclusion
In summary, achieving proficiency in AWS Lambda logging best practices stands as a critical endeavor to uphold the dependability, efficiency, and safeguarding of serverless applications residing within the Amazon Web Services ecosystem. Adhering to the recommendations elucidated within this discourse empowers both developers and operational teams to fully leverage the extensive capacities offered by AWS Lambda, all the while upholding a sturdy framework for monitoring and resolving issues.