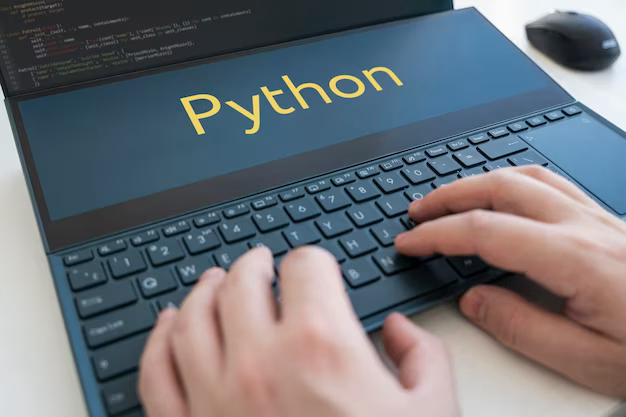
Logging stands as a pivotal facet within the realm of application development, especially in our contemporary landscape where a myriad of elements converge to form a singular solution. As the intricacies of development multiply, effective administration becomes the linchpin for ensuring the desired outcomes.
Incorporating logs during the development process serves a dual purpose: aiding in debugging and resolving application issues, while concurrently monitoring its performance throughout the developmental journey. Python offers a comprehensive logging module endowed with a plethora of widely employed logging features, significantly simplifying the task of documenting the intricacies of the development process.
Deciphering Python Logging
Python Logging functions as an event tracking mechanism, diligently monitoring an application or software’s execution. Developers employ logging as a diagnostic tool to pinpoint the root cause of issues when an application or software encounters a malfunction.
Without the aid of logging, identifying the origins of crashes or bugs becomes a Herculean task. Logging provides a trail of breadcrumbs, elucidating the sequence of glitches and mishaps leading to the primary issue precipitating crashes and other performance anomalies in the application or software.
Omitting Python logging from the equation invariably complicates the process of locating and resolving bugs or errors within the developed solution.
Each event documented through logging encapsulates variable data, potentially unique for each log entry and intricately linked to the event’s occurrence. Moreover, in Python, developers possess the ability to categorize each logged event with a specific level or severity indicator.
Navigating Python Logging
Python boasts an in-built logging module nestled within the Python Standard Library, readily accessible to developers. To initiate logging to a console, program, or file, the fundamental command entails invoking logging.basicConfig().
Within the parentheses, one must specify both the level and the message, both of which will become visible on the console and comprehensible to anyone perusing the logs at a later juncture.
The realm of logging entails the utilization of loggers, essentially entities that developers can fashion and configure to document log messages in varying formats. Loggers facilitate the organization of logging reports, offering the flexibility to redirect reports to distinct files while associating specific logging levels with particular modules. It is incumbent upon the individuals working with Python’s logging framework to possess a comprehensive grasp of the module and the associated logging levels.
The Logging Module Unveiled
The Python logging module emerges as an omnipotent tool, catering to the logging requisites of neophytes and conglomerates alike. This logging module exhibits compatibility with third-party Python libraries.
Incorporating a multitude of third-party integrations, the logging module amalgamates logs from diverse sources, creating a unified log for the entire application. This amalgamation streamlines the process of identifying errors, causative factors, and other pivotal events within the solution, rendering it more efficient, expeditious, and simplified.
Incorporating the module into one’s project is an effortless task, involving the inclusion of a succinct code fragment: import logging.
Once the logging module is assimilated, it bestows the capability to fashion and document messages, rendering them comprehensible to fellow team members. Events appended to the logger are generally categorized into five levels, each indicative of its severity. The Python logging levels encompass DEBUG, INFO, WARNING, ERROR, and CRITICAL. The nomenclature of these levels inherently conveys their significance, and the intended reader is tasked with addressing the corresponding segment of the code script augmented with the relevant level.
To gain a deeper understanding, peruse the Python logging example below:
import logging logging.debug('debug xyz')
logging.info('working as anticipated')
logging.warning('insufficient disk space')
logging.error('carousel functionality compromised')
logging.critical('unresponsive login function')
The resultant output of the above Python logging format assumes the following structure:
DEBUG:root: debug xyz
INFO:root: working as anticipated
WARNING:root: insufficient disk space
ERROR:root: carousel functionality compromised
CRITICAL:root: unresponsive login function
Ascending from Debug to Critical, the severity of each level incrementally intensifies. Developers analyzing the logs should prioritize addressing critical logs before proceeding to debug-level issues.
Fundamental Configurations
Let’s delve into a couple of configurations within Python logging, devised to ensure that log messages reach their intended destinations. The Python logging module, as previously elucidated, furnishes an array of mechanisms to accomplish this objective, assuring a straightforward configuration process.
The severity level functions—debug, info, error, warning, and critical—will automatically invoke basicConfig() when a logger lacks a defined handler. Basic configurations entail several key parameters, including level, filename, filemode, and format.
The level denotes the severity level, as detailed earlier, while the filename specifies the destination file for logging messages. Filemode dictates the mode in which the file will open, with the default mode being append. Finally, the format delineates the structure of the log message.
An exemplar of the basic configuration, including a severity level, is as follows:
logging.basicConfig(level=logging.DEBUG)
logging.debug('This message will be logged')
Crafting the Output Format
The latitude for appending strings from the extant application under construction to convey messages to the logs knows no bounds. However, it’s worth noting that certain fundamental elements have already been incorporated into the LogRecord and can be incorporated into the output format designed for Python logging messages.
Additionally, the formatter is preset for continued usage; nevertheless, the default formatter for output remains an option if needed.
The default formatter adheres to the following template:
BASIC_FORMAT = "%(levelname)s:%(name)s:%(message)s"
While this constitutes the default string, the log recorder accommodates any string that aligns with LogRecord attributes. The complete catalogue of usable attributes commences at index 1640.
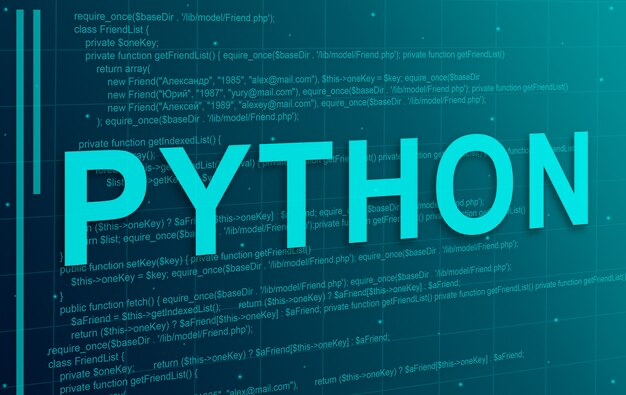
Classes and Functions
Python logging encompasses classes and functions, a crucial consideration when dealing with multifarious modules within an application—an occurrence that is the norm. It is imperative to identify these classes and functions and appreciate their significance.
The root logger serves as the default string employed in the Python logging format. Nonetheless, a concerted effort should be made to delineate one’s logger, incorporating additional classes and functions as needed.
Herein lie some commonplace strings essential for crafting appropriate log records:
- Logger: Directly employed to invoke functions within the application code;
- LogRecord: Python loggers autonomously generate LogRecord objects, provided that they possess all requisite information regarding the logged event;
- Handler: Handlers are instrumental in directing LogRecords to their intended destinations or outputs, whether it be a console or a file. Handlers form the foundation from which subclasses of strings, including StreamHandler, FileHandler, HTTPHandler, etc., can be derived;
- Formatter: The function of the Formatter is self-evident, serving as the means to define the Python logging format or specify a string conforming to a predefined format.
Implementing Python Logger
Implementing a Python logger entails leveraging the strings and commands outlined above, with the indispensable aid of the logger module. The implementation process elucidates the sequential progression of log creation and the assurance of desired outputs.
The journey commences with the creation of a logger using logger = logging.getLogger(nm), where ‘nm’ signifies the filename. Subsequently, the log-level must be retrieved from a properties file, typically formatted as .txt, and the levels must be configured therein.
Following this, the format is set using the Formatter function. Handlers are created and equipped with Formatters, culminating in the attachment of Handlers to the logger.
Creating a Log File in Python
Generating a log file in Python necessitates the inclusion of string imports, augmented with additional strings to align with specific requirements. Present-day Python developers infrequently resort to log files, opting instead for Syslog to inscribe messages into a designated file.
Creating a log file mandates crafting strings in a manner conducive to facile detection of both the file and its contained messages. For added assistance, logrotate or WatchedFileHandler can be employed to enhance event tracking and facilitate log file rotation.
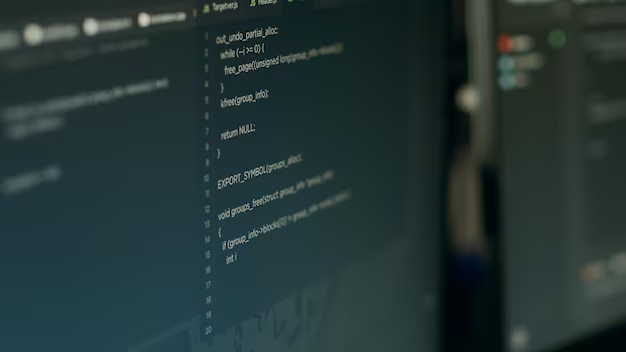
Pros and Cons of Multi-Factor Authentication
Multi-factor authentication (MFA) stands as a pivotal component of modern cybersecurity, but it carries both benefits and drawbacks. As we explore the world of Python logging, let’s intertwine this crucial aspect with the pros and cons of MFA.
Pros:
- Enhanced Security: MFA fortifies digital defenses by requiring multiple forms of identification, reducing the risk of breaches from stolen credentials;
- Robust User Verification: MFA combines what users know, have, and are, ensuring a high level of identity certainty;
- Adaptive Security: MFA systems can adapt to contextual factors, tailoring security to the situation;
- Compliance: Many industries mandate MFA, aligning with regulations and fortifying security.
Cons:
- Complexity: Implementing MFA can introduce complexity, potentially frustrating users;
- Cost: MFA methods may entail expenses for hardware or licenses;
- User Resistance: Users may find MFA inconvenient, necessitating a balance between security and usability;
- Recovery Challenges: Lost MFA devices or forgotten credentials can lead to recovery hassles;
- Phishing Vulnerabilities: MFA may not fully guard against sophisticated phishing attacks.
Integrating MFA with Python Logging
In application development, merging MFA with Python logging adds a security layer to log access. By requiring MFA authentication for log management, organizations enhance data protection. Python’s flexible logging module allows for MFA-related log integration, aiding security monitoring and compliance reporting. Balancing MFA’s strengths and weaknesses can bolster cybersecurity while preserving log integrity.
In Conclusion
Python logging represents a fundamental and universally embraced practice within the purview of Python development. It encompasses a robust logging framework complemented by an extensive repository of strings, facilitating the creation of a Python console log.
When employing the Python logger, it becomes incumbent upon developers to simplify its implementation, rendering it comprehensible to all team members.